Programming #
The NORVI EC-M11-EG-C5 has a mini USB port for serial connection with the SoC for programming. Any ESP32-supported programming IDE can be used to program the controller. Follow this Guide to programming NORVI ESP32-based Based Controllers with Arduino IDE.
SoC: ESP32-WROOM32
Programming Port: USB UART
8-pin Connector and wire harness #
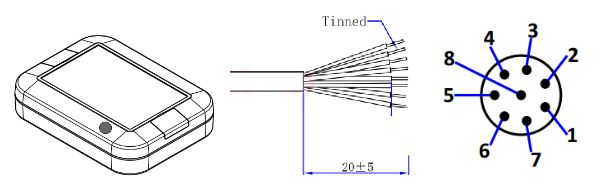
Pin Description #
8P Male | Wire color | I/O Configuration |
1 | White | SCL |
2 | Brown | SDA |
3 | Green | – |
4 | Yellow | – |
5 | Gray | 3.3V+ / 5V+ |
6 | Pink | – |
7 | Blue | Power+ |
8 | Red | Power- |
I2C Communication #
IC Type | ADS 1115 |
Module Address | 0x49 |
SDA | GPIO16 |
SCL | GPIO17 |
Programming I2C Communication #
#include <Wire.h>
// Define the I2C device address
#define DEVICE_ADDRESS 0x49
void setup() {
Wire.begin(16, 17); // SDA on GPIO16, SCL on GPIO17
Serial.begin(115200);
}
void loop() {
// Write data to the I2C device
Wire.beginTransmission(DEVICE_ADDRESS);
Wire.write(0x01); // Replace with your data
Wire.write(0x02);
Wire.write(0x03);
Wire.endTransmission();
delay(100);
// Read data from the I2C device
Wire.requestFrom(DEVICE_ADDRESS, 3); // 3 bytes of data
if (Wire.available() >= 3) {
byte data1 = Wire.read();
byte data2 = Wire.read();
byte data3 = Wire.read();
Serial.print("Read Data: ");
Serial.print(data1, HEX);
Serial.print(" ");
Serial.print(data2, HEX);
Serial.print(" ");
Serial.println(data3, HEX);
}
delay(1000); // Delay for demonstration purposes
}