Programming #
The NORVI AGENT 1-BT03-ES-L has a mini USB port for serial connection with the SoC for programming. Any ESP32-supported programming IDE can be used to program the controller. Follow this Guide to programming NORVI ESP32-based controllers with the Arduino IDE.
SoC: ESP32-WROVER-B
Programming Port: USB UART
Digital Inputs #
Wiring Digital Inputs #
The digital inputs of NORVI AGENT 1-BT03-ES-L can be configured as both sink and source connections. The inverse of the Digital Input polarity should be supplied to the common terminal.
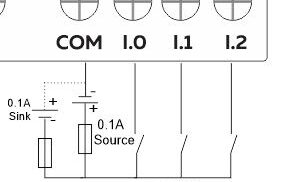
Programming Digital Inputs #
Reading the relevant GPIO of the ESP32 gives the value of the Digital Input. When the inputs are in the OFF state, the GPIO goes HIGH, and when the input is in the ON state, the GPIO goes LOW. Refer to the GPIO allocation table in the datasheet for the digital input GPIO.
#define INPUT1 21
void setup() {
Serial.begin(115200);
Serial.println("Device Starting");
pinMode(INPUT1, INPUT);
}
void loop() {
Serial.print(digitalRead(INPUT1));
Serial.println("");
delay(500);
}
Transistor Output #
Wiring Transistor Outputs #
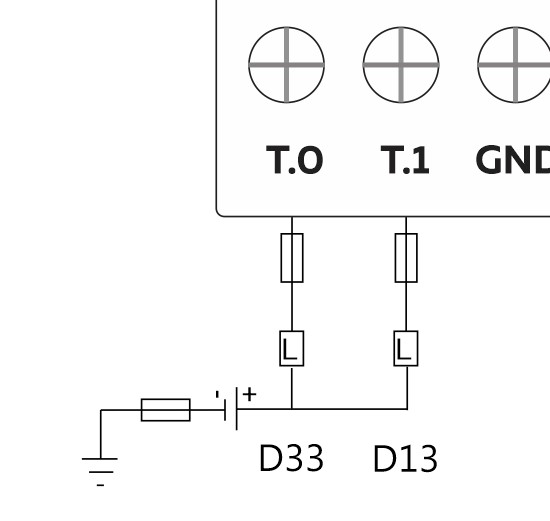
Programming Transistor Outputs #
Reading the relevant GPIO of the ESP32 gives the value of the Transistor Output. Refer to the GPIO allocation table in the datasheet for the Transistor Output GPIO.
#define OUTPUT1 33
void setup() {
Serial.begin(115200);
Serial.println("Device Starting");
pinMode(OUTPUT1 , OUTPUT);
}
void loop() {
digitalWrite(OUTPUT1, HIGH);
delay(500);
digitalWrite(OUTPUT1, LOW);
delay(500);
Serial.println("");
delay(500);
}
Thermocouple Input #
SPI MISO | GPIO19 |
SPI SCK | GPIO18 |
CS1 / Thermocouple TA | GPIO26 |
CS2 / Thermocouple TB | GPIO27 |
Programming Thermocouple Inputs #
#include <SPI.h>
#include "Adafruit_MAX31855.h"
// Example creating a thermocouple instance with software SPI on any three
// digital IO pins.
#define MAXDO 19
#define MAXCS_1 26
#define MAXCS_2 27
#define MAXCLK 18
// Initialize the Thermocouple
Adafruit_MAX31855 thermocouple_1(MAXCLK, MAXCS_1, MAXDO);
Adafruit_MAX31855 thermocouple_2(MAXCLK, MAXCS_2, MAXDO);
void setup() {
Serial.begin(115200);
Serial.println("MAX31855 test");
// Wait for MAX chips to stabilize
delay(500);
Serial.print("Initializing sensor 1...");
if (!thermocouple_1.begin()) {
Serial.println("ERROR.");
while (1) delay(10);
}
Serial.print("Initializing sensor 2...");
if (!thermocouple_2.begin()) {
Serial.println("ERROR.");
while (1) delay(10);
}
// OPTIONAL: Can configure fault checks as desired (default is ALL)
// Multiple checks can be logically OR'd together.
// thermocouple.setFaultChecks(MAX31855_FAULT_OPEN | MAX31855_FAULT_SHORT_VCC);
// Short to GND fault is ignored
Serial.println("DONE.");
}
void loop() {
double c1 = thermocouple_1.readCelsius();
double c2 = thermocouple_2.readCelsius();
if (isnan(c1)) {
Serial.println("Thermocouple 1 fault(s) detected!");
uint8_t e = thermocouple_1.readError();
if (e & MAX31855_FAULT_OPEN) Serial.println("FAULT: Thermocouple is open - no connections.");
}
else {
Serial.print("C1 = ");
Serial.println(c1);
}
if (isnan(c2)) {
Serial.println("Thermocouple 2 fault(s) detected!");
uint8_t e = thermocouple_2.readError();
if (e & MAX31855_FAULT_OPEN) Serial.println("FAULT: Thermocouple is open - no connections.");
}
else {
Serial.print("C2 = ");
Serial.println(c2);
}
delay(200);
Serial.println("");
}
Built-in Buttons #
Button 1 Pin | Digital Input GPIO35 |
GPIO Connection of buttons
Programming Buttons #
#include <Adafruit_NeoPixel.h>
#define BUTTON_PIN 35 //Digital IO pin connected to the button. This will be
#define PIXEL_PIN 25 // Digital IO pin connected to the NeoPixels.
#define PIXEL_COUNT 1
Adafruit_NeoPixel strip = Adafruit_NeoPixel(PIXEL_COUNT, PIXEL_PIN, NEO_GRB + NEO_KHZ800);
bool oldState = HIGH;
int showType = 0;
void setup() {
Serial.begin(115200);
pinMode(BUTTON_PIN, INPUT_PULLUP);
strip.begin();
strip.show(); // Initialize all pixels to 'off'
Serial.println("RS-485 TEST");
}
void loop() {
bool newState = digitalRead(BUTTON_PIN);
if (newState == LOW && oldState == HIGH) {
delay(20);
newState = digitalRead(BUTTON_PIN);
if (newState == LOW) {
showType++;
if (showType > 9)
showType = 0;
startShow(showType);
}
}
oldState = newState;
}
void startShow(int i) {
switch (i) {
case 0: colorWipe(strip.Color(0, 0, 0), 50); break;
case 1: colorWipe(strip.Color(255, 0, 0), 50); break;
case 2: colorWipe(strip.Color(0, 255, 0), 50); break;
case 3: colorWipe(strip.Color(0, 0, 255), 50); break;
case 4: theaterChase(strip.Color(127, 127, 127), 50); break;
case 5: theaterChase(strip.Color(127, 0, 0), 50); break;
case 6: theaterChase(strip.Color(0, 0, 127), 50); break;
case 7: rainbow(20); break;
case 8: rainbowCycle(20); break;
case 9: theaterChaseRainbow(50); break;
}
}
LoRaWAN #
Specification | Long Range(LoRa) |
---|---|
RF Transceiver | RFM95W-915S2 |
bit Rate | Up to 300 kbps |
Link Budget | +20 dBm – 100 mW |
RF sensitivity | -148 dBm |
SPI MISO | GPIO19 |
SPI MOSI | GPIO23 |
SPI SCK | GPIO18 |
NSS | GPIO2 |
DIO0 | GPIO4 |
DIO1 | GPIO15 |
DIO2 | NOT CONNECTED |
RESET | GPIO5 |
USB and Reset #
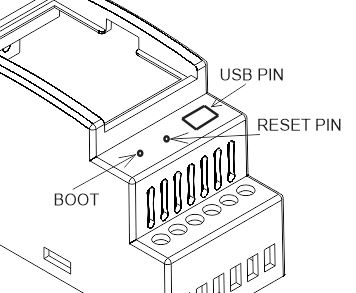